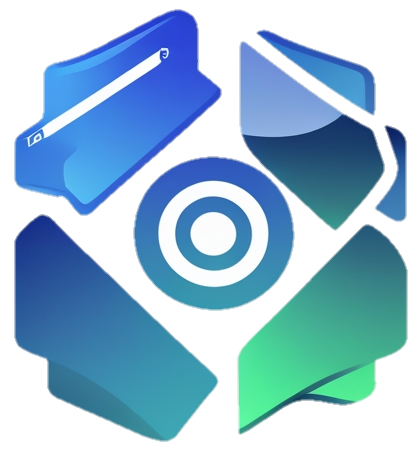
React Native Pdf From Image
Generate PDF documents from an array of images.
🌟 Features
- Image to PDF Conversion: Effortlessly convert images into PDF documents.
- Customizable Paper Sizes: Choose from standard paper sizes or define custom dimensions for your PDFs.
Old Architecture Support
react-native-pdf-from-image is a pure TurboModule, and requires the new architecture to be enabled.
- Work is ongoing to support the old architecture.
The library now supports both new and old architecture! 🎉🎉🎉
🚀 Installation
npm install react-native-pdf-from-image
or
yarn add react-native-pdf-from-image
cd ios && pod install
📖 Usage
Here's a basic example of how to use the library:
import { createPdf } from 'react-native-pdf-from-image';
const images = ['path/to/image1.jpg'];
const { filePath } = createPdf({
imagePaths: images,
name: 'myPdf',
// Optional
paperSize: 'A4',
// Optional
customPaperSize: {
height: 300,
width: 300,
},
});
Note: When using the old architecture, make sure to await the
createPdf
function as it returns a Promise.
import { createPdf } from 'react-native-pdf-from-image';
// Old Architecture
const generatePdf = async () => {
const images = ['path/to/image1.jpg'];
const { filePath } = await createPdf({
imagePaths: images,
name: 'myPdf',
// Optional
paperSize: 'A4',
// Optional
customPaperSize: {
height: 300,
width: 300,
},
});
};
📦 Props
createPdf(params)
- params : An object containing the following properties:
- imagePaths (Array of strings): An array of file paths to the images you want to include in the PDF.
- name (string): The name of the PDF file to be created.
- paperSize (string, optional): The size of the paper for the PDF. Common sizes like 'A4' are supported.
- customPaperSize (object, optional): An object specifying custom dimensions for the paper size. It should have height and width properties.
Returns
- An object containing:
- filePath (string): The file path to the generated PDF document.
Note: If neither paperSize or customPaperSize is passed then the image dimensions would be used as the paper size of the pdf.
License
This project is licensed under the MIT License.